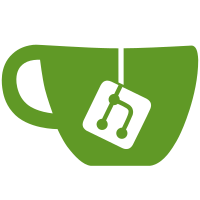
12.10.2005 - Build 12 (Eggs): - Members can now leave the Guild without requiring an officer to demote them to zero. - Rank 5 members can now edit/update Guild settings. - # of Members is now set to 1 when a Guild is created, and updated correctly when an application is approved. - The Guild founder is now notified via the Post Office when someone applies to join. - Members can now arbitrarily deposit money to the Guild bank. - You can no longer send guild money to yourself. Checks and balances, man! - Fixed image format selection so that it actually works. - Removed Language selection from account registration/settings.
49 lines
1.4 KiB
PHP
49 lines
1.4 KiB
PHP
<?php // login.php :: Handles user logins and logouts.
|
|
|
|
include("lib.php");
|
|
if (isset($_GET["do"])) { $do = $_GET["do"]; } else { $do = ""; }
|
|
|
|
switch($do) {
|
|
case "logout":
|
|
logout();
|
|
break;
|
|
default:
|
|
login();
|
|
}
|
|
|
|
function login() {
|
|
|
|
if (isset($_POST["submit"])) {
|
|
|
|
// Setup.
|
|
include("config.php");
|
|
extract($_POST);
|
|
$query = doquery("SELECT id,password FROM {{table}} WHERE username='$username' LIMIT 1", "accounts");
|
|
$row = dorow($query);
|
|
|
|
// Errors.
|
|
if ($row == false) { err("Invalid username. Please <a href=\"index.php\">go back</a> and try again.", false, false); }
|
|
if ($row["password"] != md5($password)) { err("Invalid password. Please <a href=\"index.php\">go back</a> and try again.", false, false); }
|
|
|
|
// Finish.
|
|
$newcookie = $row["id"] . " " . $username . " " . md5($row["password"] . "--" . $dbsettings["secretword"]);
|
|
if (isset($remember)) { $expiretime = time()+31536000; $newcookie .= " 1"; } else { $expiretime = 0; $newcookie .= " 0"; }
|
|
setcookie("scourge", $newcookie, $expiretime, "/", "", 0);
|
|
die(header("Location: index.php"));
|
|
|
|
} else {
|
|
|
|
display("Log In", gettemplate("login"), false);
|
|
|
|
}
|
|
|
|
}
|
|
|
|
function logout() {
|
|
|
|
setcookie("scourge", "", (time()-3600), "/", "", 0);
|
|
die(header("Location: login.php?do=login"));
|
|
|
|
}
|
|
|
|
?>
|