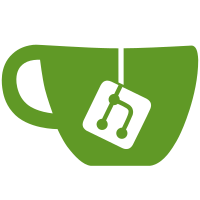
12.10.2005 - Build 12 (Eggs): - Members can now leave the Guild without requiring an officer to demote them to zero. - Rank 5 members can now edit/update Guild settings. - # of Members is now set to 1 when a Guild is created, and updated correctly when an application is approved. - The Guild founder is now notified via the Post Office when someone applies to join. - Members can now arbitrarily deposit money to the Guild bank. - You can no longer send guild money to yourself. Checks and balances, man! - Fixed image format selection so that it actually works. - Removed Language selection from account registration/settings.
64 lines
2.3 KiB
PHP
64 lines
2.3 KiB
PHP
<?php // fightmods.php :: functions for modifiers granted to you by items.
|
|
|
|
function hpleech($player) {
|
|
|
|
/***********
|
|
Description: A percentage of the final damage is given back to the player's HP.
|
|
Occurs: Per Turn.
|
|
Applies To: Player or Monster.
|
|
***********/
|
|
|
|
global $userrow, $fightrow, $monsterrow;
|
|
if ($player == "player") {
|
|
$userrow["currenthp"] += floor(($fightrow["playerphysdamage"]+$fightrow["playermagicdamage"]+$fightrow["playerfiredamage"]+$fightrow["playerlightdamage"]) * ($userrow["hpleech"]/100));
|
|
if ($userrow["currenthp"] > $userrow["maxhp"]) { $userrow["currenthp"] = $userrow["maxhp"]; }
|
|
} else {
|
|
$userrow["currentmonsterhp"] += floor(($fightrow["monsterphysdamage"]+$fightrow["monstermagicdamage"]+$fightrow["monsterfiredamage"]+$fightrow["monsterlightdamage"]) * ($monsterrow["hpleech"]/100));
|
|
if ($userrow["currentmonsterhp"] > $monsterrow["maxhp"]) { $userrow["currentmonsterhp"] = $monsterrow["maxhp"]; }
|
|
}
|
|
|
|
}
|
|
|
|
function mpleech() {
|
|
|
|
/***********
|
|
Description: A percentage of the final damage is given back to the player's MP.
|
|
Occurs: Per Turn.
|
|
Applies To: Player only.
|
|
***********/
|
|
|
|
global $userrow, $fightrow;
|
|
$userrow["currentmp"] += floor(($fightrow["playerphysdamage"]+$fightrow["playermagicdamage"]+$fightrow["playerfiredamage"]+$fightrow["playerlightdamage"]) * ($userrow["mpleech"]/100));
|
|
if ($userrow["currentmp"] > $userrow["maxmp"]) { $userrow["currentmp"] = $userrow["maxmp"]; }
|
|
|
|
}
|
|
|
|
function hpgain() {
|
|
|
|
/***********
|
|
Description: A fixed number is added to player's HP.
|
|
Occurs: Per Kill.
|
|
Applies To: Player only.
|
|
***********/
|
|
|
|
global $userrow, $fightrow;
|
|
$userrow["currenthp"] += $userrow["hpgain"];
|
|
if ($userrow["currenthp"] > $userrow["maxhp"]) { $userrow["currenthp"] = $userrow["maxhp"]; }
|
|
|
|
}
|
|
|
|
function mpgain() {
|
|
|
|
/***********
|
|
Description: A fixed number is added to player's MP.
|
|
Occurs: Per Kill.
|
|
Applies To: Player only.
|
|
***********/
|
|
|
|
global $userrow, $fightrow;
|
|
$userrow["currentmp"] += $userrow["mpgain"];
|
|
if ($userrow["currentmp"] > $userrow["maxmp"]) { $userrow["currentmp"] = $userrow["maxmp"]; }
|
|
|
|
}
|
|
|
|
?>
|